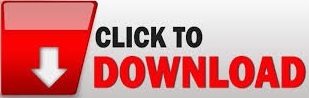
If start_index + array.length < 0, the indexing starts from index 0. If start_index<0, the indexing starts from the end of the array with the last element having the index -1. If delete_count= array.length, none of the elements will be removed, and new elements (if passed as arguments) will be added to the end of the array.
The same result occurs in the case whenĭelete_count >= (array.length - start_index).
If only the start_index is passed as a parameter, the splice() method will start removing all the elements from the start_index to the end of the array. If no elements are removed, an empty array is returned. The return value is a new array containing the deleted elements. Splice modifies the original array by removing, replacing, or adding elements. While the parameters delete_count and elements to be added are optional, the start_index is a necessary parameter. The JavaScript splice () method is a built-in method for JavaScript array functions. The splice in JavaScript removes the existing elements and adds newer ones to the array. The splice method in javascript is supported by all the browsers such as Chrome, Edge, Safari, Opera etc. In the above example, you can observe that the delete_count was 1, so the element at index 0 was removed, and new elements X, Y, Z were added. The starting point for the new elements to be added will be the start_index itself. In the coming example, we'll remove two elements from index 3 (start_index) and add two elements. Note: If no element has been removed, an empty array will be returned. The splice in JavaScript returns an array containing all the elements that have been removed from the original array. When splicing an array of objects, the data returned from the function must be cast to the object arrays data type. Return Value of Array splice() in Javascript If it has not been specified, then the splice method only removes the existing elements from the array and doesn't add any new elements. It denotes the elements that will be added right from the start_index position. javascript - Split array into chunks - Stack Overflow Split array into chunks Ask Question Asked 11 years, 6 months ago Modified 23 days ago Viewed 1.1m times 970 Let's say that I have an Javascript array looking as following: 'Element 1','Element 2','Element 3'. element1, element2., elementN (optional): Since Arrays in JavaScript are objects, it can accept elements of different data types. If not needed, the value delete_count should be 0 because if delete_count is ignored, all the elements starting from start_index are removed. The removal begins at the start_index and goes up to the number of elements as specified by the parameter, delete_count. delete_count (optional): delete_count is an integer that denotes the number of elements to be removed from the array. When it is negative, the counting would start from the end of the array starting with -1. start_index is a mandatory parameter that begins at the 0th index from the beginning of the array when it is specified to be non-negative. Start_index (required): start_index is an integer that shows the index from where the modification would take place. The splice() method accepts three parameters: And you're equipped with a handy mnemonic, that splice compared to slice has an additional letter, 'p', which helps you remember that splice mutates and optionally adds or removes from the original array.Parameters of Array splice() in Javascript
Here is the syntax of the method: slice () slice (start) slice (start, end) Let’s see these parameters.
It does not modify the original array rather returns a new array. You now know that slice makes a shallow copy of the original array, while splice mutates the original array and optionally adds or removes elements. The slice () method is used to return a new array containing a portion of that array. ConclusionĪnd there we have it! This blog goes over the differences between slice and splice. And because splice can add and remove stuff to the original array, that means that it also mutates the original array. Because of the extra letter, I associate the additional letter to splice's use of adding or removing from the original array. splice has an extra letter, 'p', compared to slice. I remember the difference between slice and splice using a mnemonic. insert 'juliet' and 'zeke' at 3rd index // returns Ĭonsole.
splice ( 3, 1, 'juliet', 'zeke' ) // remove 'harper'.